touchsweep
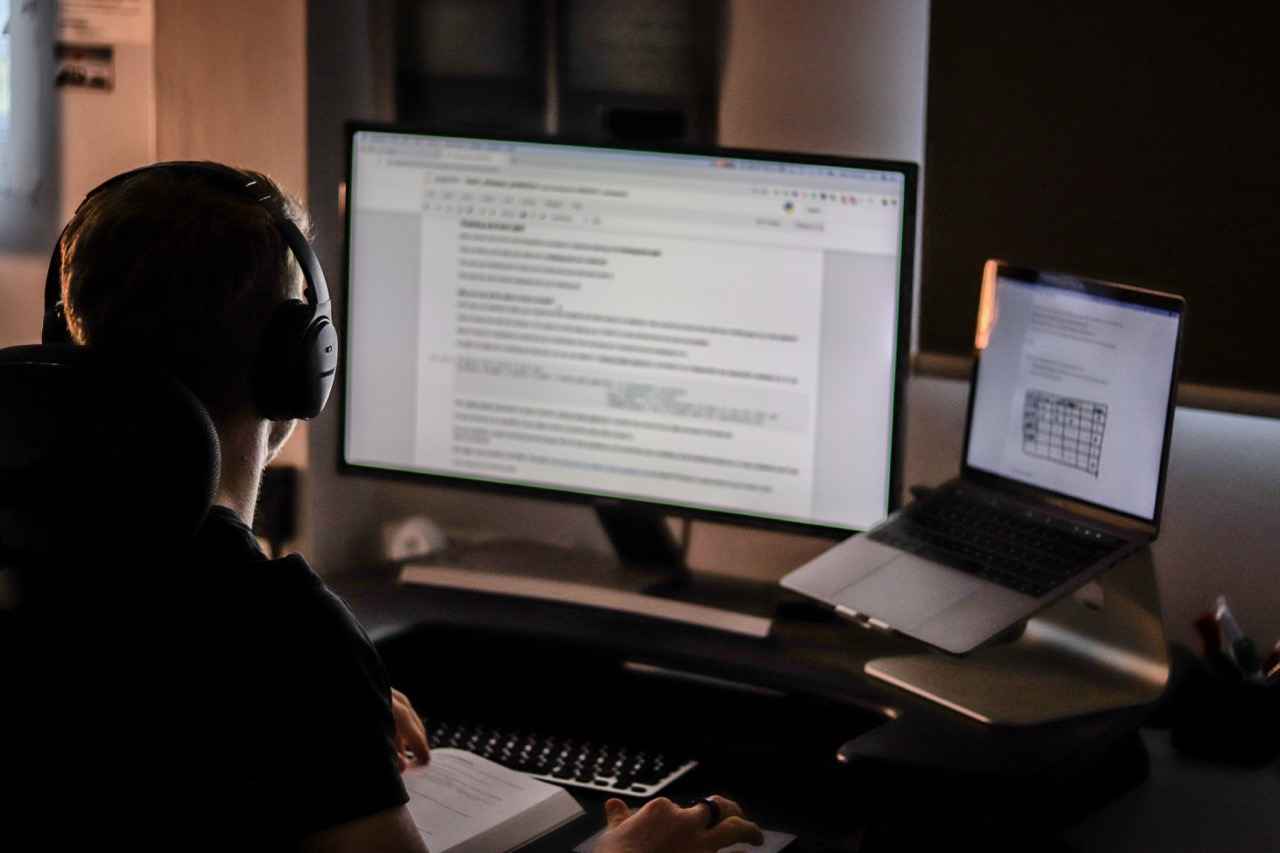
TouchSweep
Super tiny vanilla JS module to detect swipe direction and trigger custom events accordingly.
Visitor stats
Code stats
Install
npm i touchsweep
or
yarn add touchsweep
Usage
import TouchSweep from 'touchsweep';
const area = document.getElementById('swipe-area');
const data = {
value: 1
};
const touchThreshold = 20;
const touchSweepInstance = new TouchSweep(area, data, touchThreshold);
// Then listen for custom swipe events and do your magic:
area.addEventListener('swipeleft', event => {
// You have swiped left
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
area.addEventListener('swiperight', event => {
// You have swiped right
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
area.addEventListener('swipedown', event => {
// You have swiped down
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
area.addEventListener('swipeup', event => {
// You have swiped up
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
area.addEventListener('swipemove', event => {
// You are swiped continuously
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
area.addEventListener('tap', event => {
// You have tapped
// Custom event data is located in `event.detail`
// Details about coordinates are also available under `event.detail`
});
Options and default settings
The module constructor accepts three (3) arguments:
element
: A HTML Element. Default isdocument.body
eventData
: A plain JS object. Default is{}
threshold
: How many pixels to count until an event is fired. Default is 40
API
TouchSweep provides a minimal API for you to use.
The TouchSweep
instance exposes two public methods which allow you to add or to remove all event listeners responsible for the module functionality.
This is useful in cases where you want to remove the TouchSweep
container/area from the DOM and prevent possible memory leaks by removing all event listeners related to this DOM element.
In order to remove all previously attached event listeners:
touchSweepInstance.unbind();
In order to add all previously removed event listeners:
touchSweepInstance.bind();
Supported Browsers
Currently all evergreen browsers are supported.
Demo
There is a simple demo illustrating how the TouchSweep library works.
Check the code here and the live demo here
Typescript
TouchSweep
is written in Typescript and provides full Typescript support out of the box.
LICENSE
MIT