react-accordion-ts
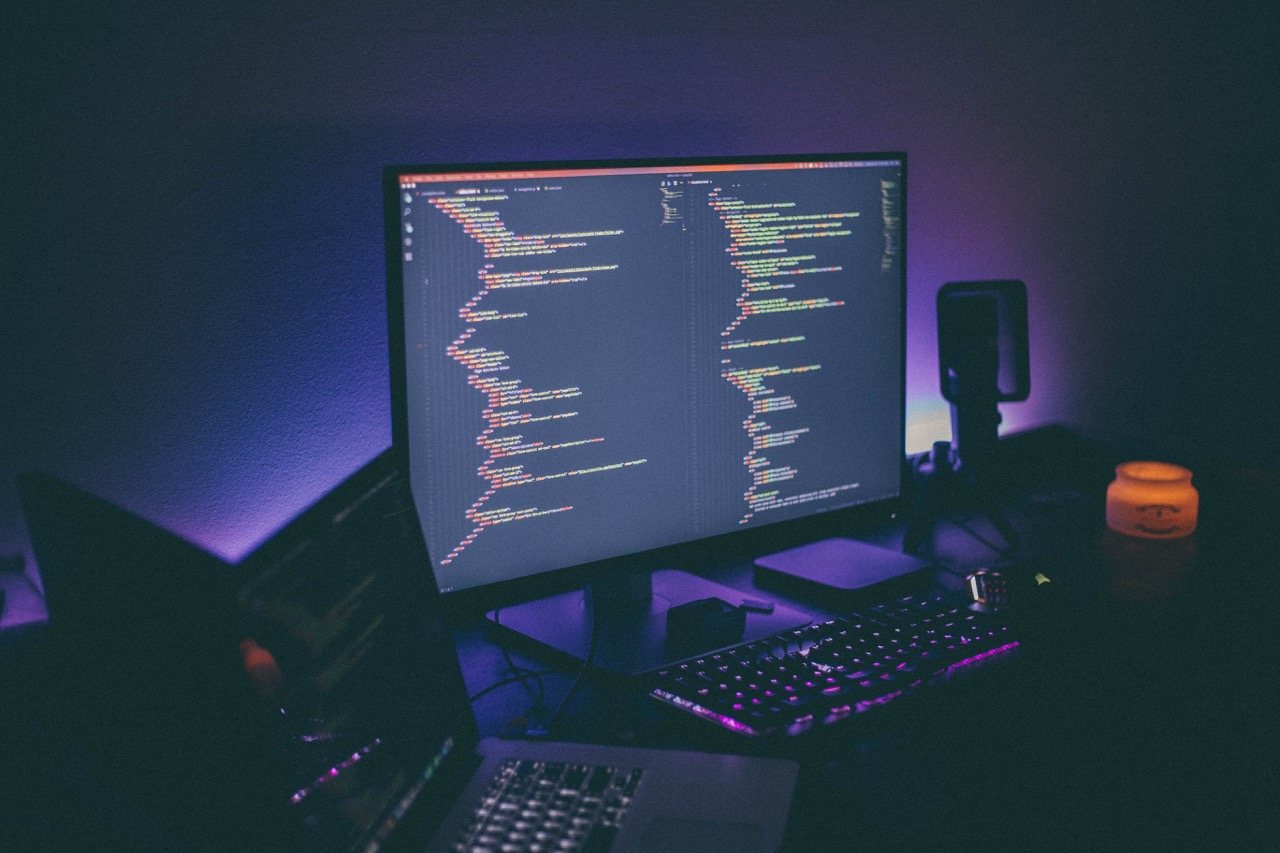
React Accordion
An accordion widget for React web applications written in Typescript.
Visitor stats
Code stats
About
This is a collapsible widget for React web applications written in TypeScript without any external dependencies (except React of course).
It uses the Hooks API and CSS to animate the collapsible contents.
The latest version of the widget has been built using the Hooks API introduced with React 16. If you need to support older versions of React, please install an older version.
Please note that this widget does NOT work with React Native.
Install
npm i react-accordion-ts
# or
yarn add react-accordion-ts
Usage
import * as React from 'react';
import { Accordion } from 'react-accordion-ts';
const content = 'Lorem ipsum, dolor sit amet consectetur adipisicing elit.';
const news = [
{
date: '12-10-2018',
title: 'Awesome title',
content: [content]
},
{
date: '13-10-2018',
title: 'Awesome title',
content: [content, content],
open: true
},
{
date: '13-10-2018',
title: 'Awesome title',
content: [content],
open: true
}
];
const items = news.map(({ open, date, title, content }) => ({
open,
title: <h2>{date + ' - ' + title}</h2>,
content: (
<>
{content.map((item: string, index: number) => (
<p key={index}>{item}</p>
))}
</>
)
}));
export const MyComponent = () => <Accordion items={items} duration={300} multiple={true} />;
You can also use the basic stylesheet included:
import 'react-accordion-ts/src/panel.css';
or
@import 'react-accordion-ts/src/panel.css';
// If the above doesn't work, add a ~ in the beginning:
@import '~react-accordion-ts/src/panel.css';
Props
Accordion props
Prop | Type | Required | Default | Description |
---|---|---|---|---|
open |
number |
false | -1 | Zero based index representing the accordion item which is initially open |
items |
AccordionItem[] |
true | - | Array of AccordionPanel objects (see below) |
duration |
number |
false | 300 | Duration (in milliseconds) of the expanding/collapsing animation |
multiple |
boolean |
false | false | A ReactNode representing the content of the slide |
Accordion panel props
Prop | Type | Required | Description |
---|---|---|---|
open |
boolean |
false | Whether the panel should be initially open |
title |
ReactNode |
true | The title of the panel. This is the element which expands/collapses the panel on click |
content |
ReactNode |
true | The panel content. |
LICENSE
MIT
Connect with me: